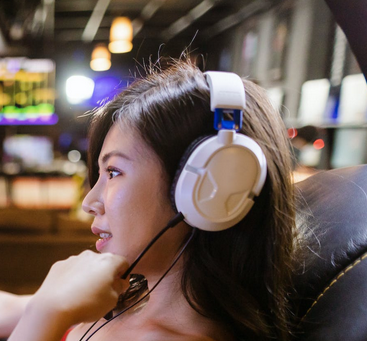
[ad_1]
💡 Downside Formulation: How does one paste a string that has been copied to the clipboard into their Python program? For example, if a consumer has copied “Good day, World!” to the clipboard, how can this system retrieve that textual content and use it inside the Python script?
Technique 1: Utilizing the Pyperclip Module
The Pyperclip module is a cross-platform Python module for copying and pasting textual content to the clipboard. It permits Python applications to work together with the clipboard, offering easy copy() and paste() features. Right here is how you need to use the paste()
perform to retrieve textual content from the clipboard.
Right here’s an instance:
import pyperclip # Assume "Good day, World!" is already copied to the clipboard copied_text = pyperclip.paste() print(copied_text)
Output:
Good day, World!
This code snippet makes use of Pyperclip’s paste()
perform to retrieve any textual content saved within the system’s clipboard. It then prints the retrieved textual content to the console.
Technique 2: Utilizing the Clipboard Module
The Clipboard module supplies a easy technique to copy and paste textual content to and from the clipboard on Home windows. For these utilizing Home windows, the clipboard module generally is a simple possibility for clipboard interactions in Python.
Right here’s an instance:
import clipboard # Assume "Good day, World!" is already copied to the clipboard copied_text = clipboard.paste() print(copied_text)
Output:
Good day, World!
The supplied instance showcases the right way to use the clipboard
module to stick textual content. The paste()
perform retrieves the content material from the clipboard and the print()
perform outputs it to the terminal.
Technique 3: Utilizing the Tkinter Module
Tkinter is the usual GUI (Graphical Consumer Interface) library for Python. Although primarily designed for creating GUIs, it additionally affords clipboard entry by way of its clipboard_get()
technique within the Tk
class.
Right here’s an instance:
import tkinter root = tkinter.Tk() root.withdraw() # Hides the Tkinter window # Assume "Good day, World!" is already copied to the clipboard copied_text = root.clipboard_get() print(copied_text) root.destroy()
Output:
Good day, World!
This snippet begins by making a hidden Tkinter window to entry the clipboard and makes use of clipboard_get()
to stick the contents. It then cleans up by destroying the Tk window after use.
Technique 4: Utilizing xclip/xsel on Linux
On Linux methods, xclip
or xsel
instructions can be utilized for clipboard operations. These instructions could be accessed from inside Python utilizing the subprocess
module.
Right here’s an instance:
import subprocess # This instance makes use of xclip. You may also use xsel by changing 'xclip' with 'xsel' within the command. copied_text = subprocess.check_output('xclip -o', shell=True).decode('utf-8') print(copied_text)
Output:
Good day, World!
This code instance leverages the xclip
command accessed by way of Python’s subprocess
module to retrieve the clipboard contents in a Linux setting.
Bonus One-Liner Technique 5: Utilizing pandas read_clipboard()
Pandas supplies a read_clipboard()
perform that can be utilized to stick tabular knowledge immediately from the clipboard right into a DataFrame. This technique is helpful for shortly importing knowledge copied from a spreadsheet or desk.
Right here’s an instance:
import pandas as pd # Assume a desk is already copied to the clipboard df = pd.read_clipboard() print(df)
Output:
Knowledge 0 Good day, World!
This one-liner code snippet exhibits the read_clipboard()
perform in motion: studying the contents of the clipboard assumed to be tabular knowledge and printing the ensuing DataFrame.
Abstract/Dialogue
- Technique 1: Pyperclip. Easy and cross-platform. Requires third-party set up. Not appropriate for pasting non-text clipboard codecs.
- Technique 2: Clipboard. Particularly for Home windows. Requires third-party module. Simple with limitations to non-text codecs and non-Home windows methods.
- Technique 3: Tkinter. A part of the Python commonplace library. GUI have to be managed, however supplies a non-third-party answer. Overkill for easy clipboard operations.
- Technique 4: xclip/xsel. Linux primarily based answer with good efficiency. Requires these utilities to be pre-installed. Not cross-platform.
- Bonus Technique 5: pandas read_clipboard(). Nice for pasting tabular knowledge. A part of the pandas library. Requires pandas and is proscribed to tabular knowledge.

Emily Rosemary Collins is a tech fanatic with a powerful background in pc science, at all times staying up-to-date with the most recent tendencies and improvements. Aside from her love for expertise, Emily enjoys exploring the nice outdoor, taking part in local people occasions, and dedicating her free time to portray and images. Her pursuits and fervour for private development make her an attractive conversationalist and a dependable supply of data within the ever-evolving world of expertise.
[ad_2]