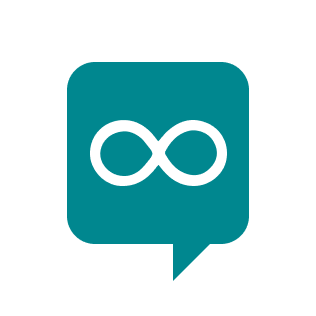
[ad_1]
I am making an attempt to amass angle values from an MPU6050 IMU sensor utilizing an ESP32-DevKitM-1 microcontroller.
My setup comprises the ESP32 board, the MPU6050 board, and an SD card reader to save lots of the readings from the sensor:
I am utilizing the Arduino IDE v2.3.0.
I tailored the next testing code excerpt from one other put up about sensor, utilizing the newer Adafruit_MPU6050 library and the SD capabilities:
#embody <Wire.h>
#embody "SPI.h"
#embody <Adafruit_Sensor.h>
#embody <Adafruit_MPU6050.h>
#embody "SD.h"
const int PIN_IMU_SCL = 22;
const int PIN_IMU_SDA = 21;
const int PIN_SD_CS = 23;
const int PIN_SD_MISO = 10;
const int PIN_SD_MOSI = 18;
const int PIN_SD_CLK = 5;
const int IMU_GyroScale = 131;
Adafruit_MPU6050 IMU;
double IMU_Time;
double IMU_TimePrev = -1;
double IMU_GyroRotX;
double IMU_GyroRotY;
double IMU_GyroRotZ;
File DataFile;
void IMU_GetData(double& angleX, double& angleY, double& angleZ, double& temp)
{
bool firstTime = false;
if (IMU_TimePrev == -1)
firstTime = true;
// Calculating the elapsed time...
IMU_TimePrev = IMU_Time;
IMU_Time = millis();
double timeDelta = (IMU_Time - IMU_TimePrev) / 1000;
// Buying the readings from the accelerometer & gyroscope...
sensors_event_t accelData;
sensors_event_t gyroData;
sensors_event_t tempData;
IMU.getEvent(&accelData, &gyroData, &tempData);
// Calculating the accelerometer angle...
double accelRotX = (180 / PI) * atan(accelData.acceleration.x / sqrt(pow(accelData.acceleration.y, 2) + pow(accelData.acceleration.z, 2)));
double accelRotY = (180 / PI) * atan(accelData.acceleration.y / sqrt(pow(accelData.acceleration.x, 2) + pow(accelData.acceleration.z, 2)));
double accelRotZ = (180 / PI) * atan(sqrt(pow(accelData.acceleration.x, 2) + pow(accelData.acceleration.y, 2)) / accelData.acceleration.z);
// Calculating the gyroscope angle...
double gyroRotX = (gyroData.gyro.x / IMU_GyroScale);
double gyroRotY = (gyroData.gyro.y / IMU_GyroScale);
double gyroRotZ = (gyroData.gyro.z / IMU_GyroScale);
// Combining the 2 (complementary filter)...
if (firstTime)
{
IMU_GyroRotX = accelRotX;
IMU_GyroRotY = accelRotY;
IMU_GyroRotZ = accelRotZ;
}
else
{
IMU_GyroRotX += timeDelta * gyroRotX;
IMU_GyroRotY += timeDelta * gyroRotY;
IMU_GyroRotZ += timeDelta * gyroRotZ;
}
angleX = (0.96 * accelRotX) + (0.04 * IMU_GyroRotX);
angleY = (0.96 * accelRotY) + (0.04 * IMU_GyroRotY);
angleZ = (0.96 * accelRotZ) + (0.04 * IMU_GyroRotZ);
temp = tempData.temperature;
}
bool IMU_Init()
{
bool standing = IMU.start();
if (standing)
{
IMU.setAccelerometerRange(MPU6050_RANGE_8_G);
IMU.setGyroRange(MPU6050_RANGE_500_DEG);
IMU.setFilterBandwidth(MPU6050_BAND_21_HZ);
}
IMU_Time = millis();
delay(100);
return standing;
}
void setup()
{
Serial.start(9600);
Serial.println("Initializing...");
Wire.start(PIN_IMU_SDA, PIN_IMU_SCL);
IMU_Init();
SPI.start(PIN_SD_CLK, PIN_SD_MISO, PIN_SD_MOSI, PIN_SD_CS);
whereas (!SD.start(PIN_SD_CS))
{
Serial.println("Unable to open SD!");
delay(500);
}
DataFile = SD.open("/angles.txt", FILE_WRITE);
DataFile.shut();
delay(100);
Serial.println("Initialized!");
}
void loop()
{
double angleX;
double angleY;
double angleZ;
double temp;
IMU_GetData(angleX, angleY, angleZ, temp);
String anglesStr = String(angleX) + ":" + String(angleY) + ":" + String(angleZ);
DataFile = SD.open("/angles.txt", FILE_APPEND);
DataFile.println(anglesStr);
DataFile.shut();
Serial.println(anglesStr);
delay(200);
}
The place IMU_GetData
is the operate liable for buying the angle information from the gyroscope and the accelerator sensors on the MPU6050 board, and implementing a filter to achieve a set of filtered angle values.
I then merely save the filtered angle values to the SD card. I talk with the sensor utilizing the Wire I2C library, and with the SD card utilizing the SPI library.
The principle difficulty I am working into is the truth that the resultant angle values don’t seem to precisely signify the rotation of the sensor.
As a take a look at, I rotated the sensor 360° slowly round every one of many 3 axes, and saved the continual angle readings throughout every rotation:
The time interval between every studying within the plots above is ~0.2 s.
The primary difficulty is {that a} rotation in regards to the Z-axis seen within the final graph above seems to not trigger any considerable change in any of the angle readings.
I initally assumed that could possibly be brought on by the worth of the IMU_GyroScale
fixed that the gyroscope angle values get multiplied by in the course of the calculations carried out within the IMU_GetData
operate. I assumed that every one MPU6050 chips will use that very same scaling issue, however then I attempted a number of of the opposite listed scaling components listed within the datasheet, with no considerable distinction.
Is there one thing visibly flawed in my code with the angle calculations for the Z-axis? May this be an indication of a faulty sensor board?
The second factor I used to be inquisitive about was the format of the filtered angle values.
I initially assumed that the filtered angle values I’d be getting from the sensor could be the Euler angles; Roll, Pitch & Yaw. Nevertheless, these seem to not signify these.
Actually, one of many predominant sources listed within the authentic posting that describes intimately the method of calculating the filtered angle values, additionally supplies a visualization of the angle values acquired from the sensor:
Are these “tilt” values associated to Euler angles? Is it doable to transform between the 2?
[ad_2]