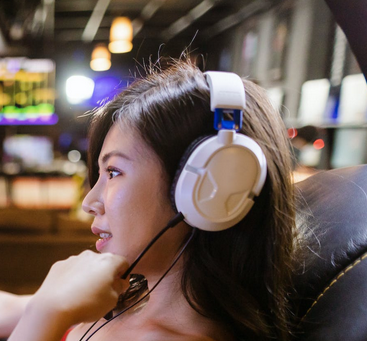
[ad_1]
💡 Downside Formulation: In Python, it’s frequent to come across a state of affairs the place you might want to examine if a string variable is None earlier than continuing with additional operations. Correct dealing with of such instances is important to keep away from bugs and errors in your code.
As an example, given a variable my_str
, you need to decide if it holds a string worth or whether it is None, which could signify the absence of a worth or an uninitialized variable.
Methodology 1: Utilizing a Easy Conditional Test
One primary methodology for checking if a string is None is by utilizing a easy conditional examine. It’s easy and readable. It straight compares the variable to None utilizing the equality (==) operator to examine if there’s an absence of a worth.
Right here’s an instance:
my_str = None if my_str is None: print("The string is None!") else: print("The string incorporates:", my_str)
Output:
🖨️ Beneficial Academy Course: Voice-First Growth: Constructing Reducing-Edge Python Apps Powered By OpenAI Whisper ⭐⭐⭐⭐⭐
The string is None!
This code snippet clearly demonstrates easy methods to use a conditional assertion to examine for None. If the situation is met, it prints a message stating that the string is None. In any other case, it prints the string’s content material. It’s a direct and user-friendly method to deal with None checks.
Methodology 2: Utilizing the ‘is’ Operator
The ‘is’ operator in Python is commonly used for evaluating identities, which makes it notably appropriate for checking if a variable is None since there’s just one occasion of None in a Python runtime.
Right here’s an instance:
my_str = None if my_str is None: print("Yep, it is None!") else: print("Nope, it has a worth!")
Output:
Yep, it is None!
By utilizing the ‘is’ operator, this snippet checks the id of my_str
, not simply its worth. If my_str
is certainly None, the message confirms it’s None. This operator gives readability in code when checking for None.
Methodology 3: Exploiting the Boolean Context of None
None in Python is taken into account False in a boolean context. By exploiting this property, you’ll be able to write a concise examine that doesn’t require a comparability operator in any respect.
Right here’s an instance:
my_str = None if not my_str: print("String might be None or empty!") else: print("String has a truthy worth!")
Output:
String might be None or empty!
This code makes use of the truth that None evaluates to False. Subsequently, the if not my_str
situation will succeed if my_str
is both None or an empty string. It’s a succinct method to examine None however lacks specificity, mistaking empty strings for None.
Methodology 4: Combining ‘is None’ with ‘or’ Operator
At instances, it’s not sufficient to examine if a string is solely None; you may also need to examine if it’s empty. Combining ‘is None’ with ‘or’ means that you can deal with each None values and empty strings in a single go.
Right here’s an instance:
my_str = None if my_str is None or my_str == "": print("The string is both None or empty.") else: print("The string has characters.")
Output:
The string is both None or empty.
This snippet makes use of logical ‘or’ to examine for a number of circumstances directly. If my_str
is None or an empty string, it prints an acceptable message. This variation permits for extra complete checking with a slight improve in verbosity.
Bonus One-Liner Methodology 5: Using a Ternary Operator
The ternary operator gives a one-liner resolution to elegantly carry out a None examine whereas assigning a worth or dealing with the variable accordingly.
Right here’s an instance:
my_str = None consequence = "No worth" if my_str is None else "Has worth" print(consequence)
Output:
No worth
This compact code makes use of a ternary operator to examine for None and assign a message to the consequence
variable primarily based on my_str
‘s worth. It’s a clear and concise method to deal with None checks inline and is especially helpful when a default worth is required.
Abstract/Dialogue
- Methodology 1: Easy Conditional Test. Simple to learn and perceive. Doesn’t cowl empty strings until particularly included within the situation.
- Methodology 2: ‘is’ Operator. Pythonic and simple. Ensures the checked worth is None and never simply falsy like an empty string or zero.
- Methodology 3: Boolean Context. Very concise. Nonetheless, it could result in false positives with empty strings or zero values, as these are additionally evaluated as False.
- Methodology 4: ‘is None’ with ‘or’. Thorough in dealing with each None and empty strings. Barely extra verbose than the opposite strategies.
- Methodology 5: Ternary Operator. Compact and stylish for inline checks and assignments. Presents readability with the trade-off of being much less specific within the None examine semantics.

Emily Rosemary Collins is a tech fanatic with a robust background in laptop science, at all times staying up-to-date with the newest traits and improvements. Other than her love for know-how, Emily enjoys exploring the nice outdoor, taking part in local people occasions, and dedicating her free time to portray and images. Her pursuits and keenness for private progress make her an interesting conversationalist and a dependable supply of data within the ever-evolving world of know-how.
[ad_2]