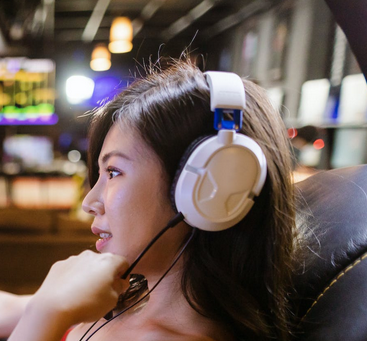
[ad_1]
💡 Downside Formulation: Changing a Python set to a string is a standard requirement when it’s worthwhile to show the contents of a set textually, log the set values right into a file, or carry out additional string manipulations. Suppose you have got a set {'apple', 'banana', 'cherry'}
and also you wish to acquire a single string that represents this set, resembling "apple banana cherry"
. This text discusses numerous strategies to realize this transformation effectively.
Technique 1: Utilizing the be a part of()
methodology
This methodology entails changing all parts of a set to a string after which becoming a member of them right into a single string with a specified separator. The be a part of()
methodology in Python takes an iterable as an argument, converts every aspect to a string, and concatenates them collectively, separated by the string on which it’s referred to as.
Right here’s an instance:
fruits_set = {'apple', 'banana', 'cherry'} fruits_string = ' '.be a part of(fruits_set) print(fruits_string)
Output:
apple banana cherry
Discover that on this code snippet, we use an area because the separator for be a part of()
, leading to a space-separated string that accommodates all the weather from the set. The order of parts within the ensuing string might fluctuate since units don’t keep order.
Technique 2: Utilizing a for loop
This methodology entails iterating over every aspect within the set, changing every to a string, and including it to an initially empty string. It’s a extra handbook method however permits for added customization throughout the conversion.
Right here’s an instance:
fruits_set = {'apple', 'banana', 'cherry'} fruits_string = '' for fruit in fruits_set: fruits_string += fruit + ' ' fruits_string = fruits_string.strip() # Eradicating the final area print(fruits_string)
Output:
apple banana cherry
Right here, we iterate over the set and concatenate every aspect to the fruits_string
with an area. Lastly, we take away the trailing area utilizing the strip()
methodology earlier than printing the ensuing string.
Technique 3: Utilizing the str()
methodology and string slicing
Utilizing Python’s built-in str()
methodology can shortly convert the set to a string, after which string slicing can clear up undesirable characters like braces.
Right here’s an instance:
fruits_set = {'apple', 'banana', 'cherry'} fruits_string = str(fruits_set)[1:-1].exchange("'", "") print(fruits_string)
Output:
apple, banana, cherry
The str()
methodology converts the set to a string with its regular illustration, together with the curly braces and quotes. Then, string slicing removes the braces, and exchange()
is used to do away with single quotes round every aspect.
Technique 4: Utilizing Listing Comprehension with be a part of()
A Python record comprehension can be utilized to transform every aspect within the set to a string, adopted by becoming a member of them utilizing the be a part of()
methodology.
Right here’s an instance:
fruits_set = {'apple', 'banana', 'cherry'} fruits_string = ' '.be a part of([str(fruit) for fruit in fruits_set]) print(fruits_string)
Output:
apple banana cherry
On this instance, we assemble an inventory of string parts utilizing an inventory comprehension after which be a part of the record parts into one string. It’s a chic one-liner that emphasizes Python’s expressive energy.
Bonus One-Liner Technique 5: Utilizing map()
with be a part of()
The map()
perform applies the str()
perform to each merchandise of the set. Then, be a part of()
is used to create the string from the map object.
Right here’s an instance:
fruits_set = {'apple', 'banana', 'cherry'} fruits_string = ' '.be a part of(map(str, fruits_set)) print(fruits_string)
Output:
apple banana cherry
The map(str, fruits_set)
name converts all set parts to strings, and be a part of()
consolidates them right into a single string, separated by an area. This methodology is environment friendly and concise.
Abstract/Dialogue
Right here’s a fast recap of the strategies mentioned:
- Technique 1: Utilizing
be a part of()
. Strengths: Easy and Pythonic. Weaknesses: Assumes all parts of the set are of a stringifiable kind. - Technique 2: Utilizing a for loop. Strengths: Presents customized aspect processing. Weaknesses: Extra verbose and probably slower for giant units.
- Technique 3: Utilizing
str()
and slicing. Strengths: Fast for small units. Weaknesses: Inelegant, with potential pitfalls on ill-formatted strings. - Technique 4: Listing Comprehension with
be a part of()
. Strengths: Elegant and versatile. Weaknesses: Requires creation of an intermediate record. - Technique 5: Utilizing
map()
withbe a part of()
. Strengths: Clear and useful programming model. Weaknesses: Will be much less readable for these unfamiliar withmap()
.

Emily Rosemary Collins is a tech fanatic with a robust background in pc science, all the time staying up-to-date with the most recent tendencies and improvements. Aside from her love for know-how, Emily enjoys exploring the good open air, taking part in area people occasions, and dedicating her free time to portray and pictures. Her pursuits and fervour for private progress make her an interesting conversationalist and a dependable supply of information within the ever-evolving world of know-how.
[ad_2]