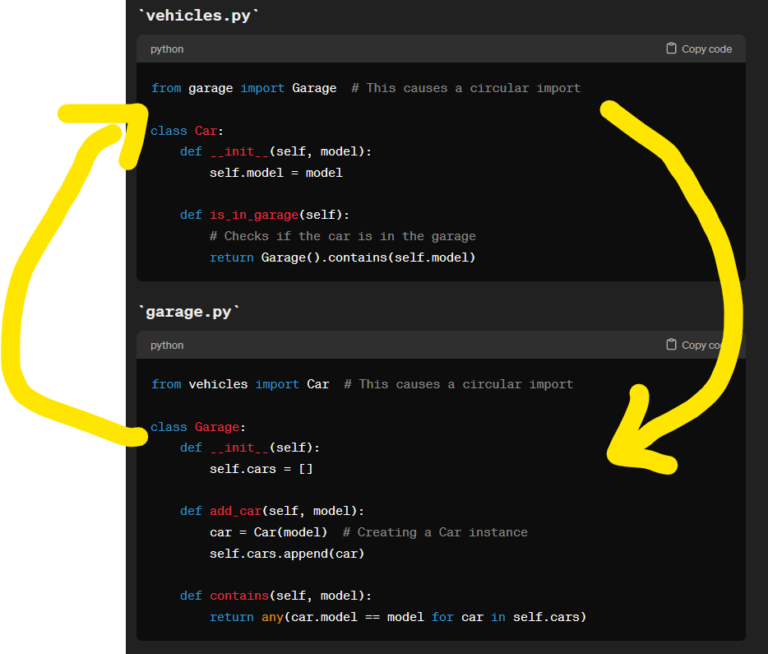
[ad_1]
Think about you and your pal try to resolve who goes by way of the door first. You say, “After you,” and your pal says, “No, no, after you.” And there you each stand… perpetually.
In Python, this occurs when Module A wants one thing from Module B, however Module B additionally wants one thing from Module A. It’s a standoff, and no person wins.
Instance of Round Import

💡 Instance: Think about you’ve gotten two Python recordsdata: autos.py
and storage.py
. In autos.py
, you outline a category Automotive
that should examine if a given automotive is presently saved in a storage from storage.py
.
Conversely, storage.py
defines a category Storage
that shops automobiles, and for some performance, it must create automotive cases utilizing the Automotive
class outlined in autos.py
. If each recordsdata try to import one another on the prime, Python will stumble right into a round import.
While you attempt to run one of many modules, Python will attempt to load autos.py
, which in flip tries to load storage.py
earlier than it has completed loading autos.py
, resulting in errors or surprising conduct as a result of one module is making an attempt to make use of components of the opposite earlier than they’re absolutely obtainable.
As an example the round import problem with a technical instance, let’s create the code for the autos.py
and storage.py
situation talked about earlier.
autos.py
from storage import Storage # This causes a round import class Automotive: def __init__(self, mannequin): self.mannequin = mannequin def is_in_garage(self): # Checks if the automotive is within the storage return Storage().comprises(self.mannequin)
storage.py
from autos import Automotive # This causes a round import class Storage: def __init__(self): self.automobiles = [] def add_car(self, mannequin): automotive = Automotive(mannequin) # Making a Automotive occasion self.automobiles.append(automotive) def comprises(self, mannequin): return any(automotive.mannequin == mannequin for automotive in self.automobiles)
On this situation, autos.py
imports Storage
from storage.py
on the prime stage, and storage.py
does the identical with Automotive
from autos.py
, making a round import drawback. When both autos.py
or storage.py
is run, Python encounters an import assertion that leads it right into a loop, inflicting errors as a result of it tries to entry performance from a module that has not been absolutely loaded but.
Normal Answer Technique
To resolve the round import problem in our autos.py
and storage.py
instance, one efficient resolution is to maneuver the import statements contained in the features or strategies the place they’re truly wanted. This fashion, the import occurs at runtime, when the perform is named, fairly than on the module’s load time, thereby avoiding the round dependency drawback. This technique is especially helpful when the imported performance shouldn’t be required instantly when the module is loaded however fairly at a particular level throughout execution, akin to inside a technique’s physique.
Fastened autos.py
# Eliminated the import from the highest to inside the tactic class Automotive: def __init__(self, mannequin): self.mannequin = mannequin def is_in_garage(self): from storage import Storage # Import moved inside the tactic return Storage().comprises(self.mannequin)
Fastened storage.py
# Saved the import of Automotive inside the tactic that wants it class Storage: def __init__(self): self.automobiles = [] def add_car(self, mannequin): from autos import Automotive # Import moved inside the tactic automotive = Automotive(mannequin) # Making a Automotive occasion self.automobiles.append(automotive) def comprises(self, mannequin): return any(automotive.mannequin == mannequin for automotive in self.automobiles)
With this method, the round import is resolved as a result of Storage
is just imported inside Automotive.is_in_garage()
when it’s wanted, and equally, Automotive
is imported inside Storage.add_car()
solely on the level of use. This ensures that each lessons could be outlined with out requiring one another on the prime stage, thus avoiding the loop of imports.
Let’s take a look at a number of (various) resolution strategies subsequent! 👇👇👇
Technique 1: Merge Collectively
If Module A and Module B are inseparable, why not mix them into one huge Module C? It simplifies issues!
Instance:
# Earlier than: A.py and B.py are two separate recordsdata inflicting points. # After: Mix into C.py the place every part lives fortunately ever after.
Technique 2: Take Turns
What when you took turns? Transfer the import inside a perform. This fashion, the code solely tries to import when the perform is named, doubtlessly avoiding the standoff.
Instance:
# In A.py def dance_with_b(): from B import b_dance b_dance()
Technique 3: Change the Routine
Generally, the dance steps are too sophisticated. Possibly Module A and Module B don’t should be so depending on one another. Cut up them up or reorganize the steps in order that they circulate in a single course.
Instance:
# As an alternative of A importing B and B importing A, # Cut up B into B1 and B2 the place B1 can safely import A and B2 doesn't.
Technique 4: Use a Choreographer 📝
Packages in Python can use an __init__.py
file to handle imports like a choreographer managing dancers. It will probably assist arrange and management how modules work together with one another.
Instance:
# Inside your package deal's __init__.py from .A import A from .B import B
Technique 5: Dance by Proxy 🎭
Inversion of Management (IoC) is like hiring a dance proxy. As an alternative of Module A and B instantly interacting, they move messages or objects by way of an middleman. It’s a bit like sending love letters as an alternative of speaking instantly.
Instance:
# A and B talk by way of an middleman, avoiding direct imports.
Technique 6: Signal Language 🤟
Summary Base Courses (ABCs) work like signal language for modules. They outline a standard language (interface) that each modules can perceive with out instantly speaking.
Instance:
# ABCs.py defines a standard interface. # A.py and B.py each import ABCs.py however not one another.
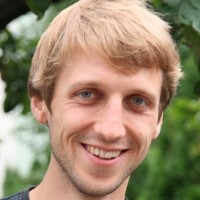
Whereas working as a researcher in distributed techniques, Dr. Christian Mayer discovered his love for educating laptop science college students.
To assist college students attain greater ranges of Python success, he based the programming training web site Finxter.com that has taught exponential expertise to hundreds of thousands of coders worldwide. He’s the writer of the best-selling programming books Python One-Liners (NoStarch 2020), The Artwork of Clear Code (NoStarch 2022), and The Guide of Sprint (NoStarch 2022). Chris additionally coauthored the Espresso Break Python collection of self-published books. He’s a pc science fanatic, freelancer, and proprietor of one of many prime 10 largest Python blogs worldwide.
His passions are writing, studying, and coding. However his best ardour is to serve aspiring coders by way of Finxter and assist them to spice up their expertise. You’ll be able to be part of his free e-mail academy right here.
[ad_2]