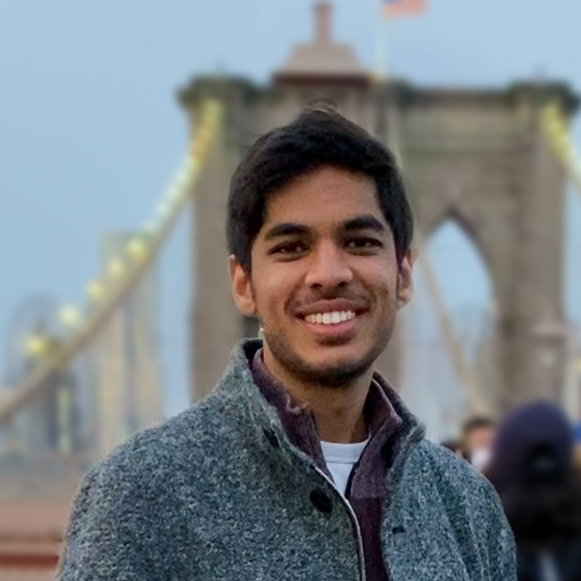
[ad_1]
How’s everybody? I’m again with one other tip/gotcha which could journey newbie programmers. This one pertains to Python scopes so if you’re already acquainted with them then this text won’t be very informative for you. In case you are not very effectively versed in Python scopes then maintain studying.
Lets begin with a code pattern. Save the next code in a file and run it:
command = "You're a LOVELY individual!"
def shout():
print(command)
shout()
print(command)
Output:
You're a LOVELY individual!
You're a LOVELY individual!
Good. Working as anticipated. Now modify it a bit and run the modified code:
command = "You're a LOVELY individual!"
def shout():
command = "HI!"
print(command)
shout()
print(command)
Output:
HI!
You're a LOVELY individual!
Wonderful! Nonetheless working high-quality. Now modify it a tad bit extra and run the brand new code:
command = "You're a LOVELY individual!"
def shout():
command = "HI!"
print(command)
command = "You might be wonderful!!"
shout()
print(command)
Output:
HI!
You're a LOVELY individual!
Umm the output shouldn’t be as intuitively anticipated however lets make one final change earlier than we talk about it:
command = "You're a LOVELY individual!"
def shout():
print(command)
command = "You might be wonderful!!"
shout()
print(command)
Output:
Traceback (most up-to-date name final):
File "prog.py", line 8, in <module>
shout()
File "prog.py", line 4, in shout
print(command)
UnboundLocalError: native variable 'command' referenced earlier than project
Woah! What’s that? We do have command declared and initialised within the very first line of our file. This would possibly stump a whole lot of newbie Python programmers. As soon as I used to be additionally confused about what was taking place. Nonetheless, if you’re conscious of how Python handles variable scopes then this shouldn’t be new for you.
Within the final instance which labored high-quality, a whole lot of learners may need anticipated the output to be:
HI!
You might be wonderful!!
The rationale for anticipating that output is easy. We’re modifying the command
variable and giving it the worth “You might be wonderful!!” throughout the perform. It doesn’t work as anticipated as a result of we’re modifying the worth of command
within the scope of the shout
perform. The modification stays inside that perform. As quickly as we get out of that perform into the international
scope, command
factors to its earlier international
worth.
After we are accessing the worth of a variable inside a perform and that variable shouldn’t be outlined in that perform, Python assumes that we need to entry the worth of a world variable with that identify. That’s the reason this piece of code works:
command = "You're a LOVELY individual!"
def shout():
print(command)
shout()
print(command)
Nonetheless, if we modify the worth of the variable or change its project in an ambiguous approach, Python provides us an error. Take a look at this earlier code:
command = "You're a LOVELY individual!"
def shout():
print(command)
command = "You might be wonderful!!"
shout()
print(command)
The issue arises when Python searches for command
within the scope of shout
and finds it declared and initialized AFTER we are attempting to print its worth. At that second, Python doesn’t know which command
‘s worth we need to print.
We will repair this drawback by explicitly telling Python that we need to print the worth of the worldwide command
and we need to edit that international variable. Edit your code like this:
command = "You're a LOVELY individual!"
def shout():
international command
print(command)
command = "You might be wonderful!!"
shout()
print(command)
Output:
You're a LOVELY individual!
You might be wonderful!!
Usually, I strive as a lot as doable to steer clear of international variables as a result of when you aren’t cautious then your code would possibly provide you with surprising outputs. Solely use international when that you would be able to’t get away with utilizing return values and sophistication variables.
Now, you would possibly ask your self why does Python “assume” that we’re referring to the worldwide variable as an alternative of throwing an error at any time when a variable isn’t outlined within the perform scope? Python docs give an attractive clarification:
What are the foundations for native and international variables in Python?
In Python, variables which are solely referenced inside a perform are implicitly international. If a variable is assigned a price anyplace throughout the perform’s physique, it’s assumed to be a neighborhood until explicitly declared as international.
Although a bit stunning at first, a second’s consideration explains this. On one hand, requiring international for assigned variables gives a bar towards unintended side-effects. Alternatively, if international was required for all international references, you’d be utilizing international on a regular basis. You’d should declare as international each reference to a built-in perform or to a part of an imported module. This muddle would defeat the usefulness of the worldwide declaration for figuring out side-effects.
I hope this was informative for you. Let me know in case you have ever confronted this concern earlier than within the feedback beneath.
[ad_2]