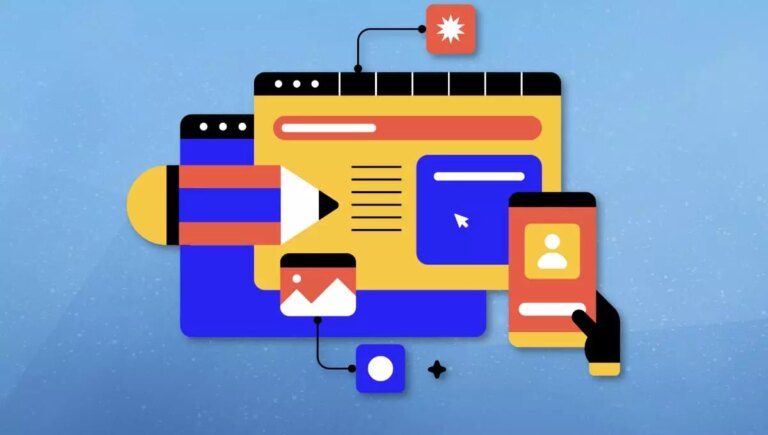
[ad_1]
On this article, we’ll introduce .NET Blazor, a strong framework that unifies client-side and server-side improvement paradigms and gives enhanced efficiency and improved tooling.
Introducing .NET Blazor
One of many fundamental challenges builders face typically is that they should know two completely different languages — one for the server-side improvement, and one for the client-side improvement. .NET Blazor tries to bridge the hole between client-side and server-side improvement by enabling builders to construct interactive net purposes utilizing C# and .NET. So builders can depend on a single improvement language framework and reuse the expertise and information they have already got.
This subject was the principle motive behind Microsoft’s .NET Blazor framework. It truly began as a private side-project of Steven Sanderson, Principal Software program Engineering Lead at Microsoft in 2017, which developed into server-side Blazor mid 2019, and client-side (WebAssembly) in 2020.
On this article, we’ll begin from the latest historical past of net app improvement, and the principle variations between server-side and client-side net app architectures. From there, we’ll map each architectures with present corresponding Blazor internet hosting fashions. Within the second a part of the article, we’ll be taught concerning the upcoming adjustments within the subsequent Blazor launch primarily based on .NET 8 later in 2023, and methods to develop our first Blazor app utilizing the present .NET 8 preview 7.
The Latest Historical past of Net App Improvement
The world of net app improvement might be summarized in server-side and client-side architectures.
Server-side, because the title implies, requires an underlying net server, akin to Home windows Web Data Server (IIS), Linux Apache, or NGINX, or a containerized platform model of the identical.
Server-side improvement depends on producing HTML on the server and sending it to the shopper. Applied sciences like ASP.NET Net Varieties present wealthy controls and abstractions, making it simpler to construct complicated net purposes. Nonetheless, the tight coupling between server and shopper typically results in challenges in sustaining scalability and responsiveness. If the shopper can’t attain the server, there’s no net web page (or a web page saying HTTP 404 error). Varied languages are used for the precise net utility improvement, akin to C# .NET, Java, and PHP.
Shopper-side refers to an online utility situation, which doesn’t require a server within the backend, however moderately runs fully within the browser.
The evolution of net app improvement started with static HTML pages. Because the demand for dynamic content material and interactivity grew, applied sciences like JavaScript emerged, enabling builders to construct extra interactive net purposes. The early 2000s witnessed the rise of AJAX (Asynchronous JavaScript and XML), which allowed for asynchronous knowledge trade between the shopper and the server, leading to smoother consumer experiences. Frameworks like jQuery additional simplified client-side scripting. 20 years later, the web sites we’re visiting day by day are nonetheless based totally on HTML and JavaScript.
Blazor Internet hosting Fashions
.NET Blazor gives two fundamental internet hosting fashions: Blazor Server and Blazor WebAssembly.
Blazor Server permits builders to create wealthy and dynamic net purposes, the place the consumer interface logic is 100% executed on the server, and the UI updates get despatched to the shopper over a persistent SignalR connection. This mannequin supplies real-time interactivity whereas sustaining a well-recognized programming mannequin for .NET builders. It’s properly suited to purposes that require dynamic updates akin to pulling up database info in a retail/eshop situation, buyer profiles, monetary inventory reporting and the like, in addition to usually having a decrease latency tolerance.
The picture beneath presents a diagram of Blazor server structure.
Let’s have a look at among the execs and cons of server-side Blazor.
Execs:
- Quick load time, assuming the online server is sized accurately.
- Closest to conventional ASP.NET Core improvement.
- Assist for older browsers, as no requirement for WebAssembly (though this might be seen as a destructive level from a safety and supportability perspective).
Cons:
- Shopper/Browser consumes extra reminiscence to run the online app, and is 100% depending on the SignalR connection.
- Every shopper session consumes CPU and reminiscence on the server, which could herald right-size challenges for purposes below heavy or spike load.
- Shopper <-> Server communication assumes a “sturdy” connection to keep away from latency and errors.
Blazor WebAssembly takes a completely completely different method, permitting builders to run .NET code straight within the browser utilizing WebAssembly (aka Wasm), a binary instruction format for net purposes. This mannequin permits Blazor to run the execution of C# code on the shopper aspect, decreasing the necessity for fixed communication with the server. Blazor WebAssembly is good for situations the place purposes must be absolutely client-side, but nonetheless offering a wealthy and responsive consumer expertise — just like what customers usually anticipate from server purposes.
The picture beneath presents a diagram of Blazor WebAssembly structure.
Let’s have a look at among the execs and cons of WebAssembly.
Execs:
- An internet site might be deployed as static information, because it all runs within the browser.
- Wasm Apps can run offline, as there is no such thing as a want for server connection (on a regular basis).
- Assist for progressive net apps (PWAs), which suggests it could act as a regionally put in utility on the shopper machine.
Cons:
- Because the JavaScript engine within the browser must obtain the complete Blazor app and corresponding .NET DLLs, the primary load of the app might be thought-about comparatively sluggish.
- WebAssembly requires a contemporary browser. If outdated browsers are nonetheless in use and required, you may solely use Blazor Server.
- If put in as a PWA, there’s a problem round model updates and administration.
- Code and DLLs might be decrypted. Any secrets and techniques akin to connection strings, passwords and the like shouldn’t be used inside the code, as they’re seen within the browser dev instruments.
Observe: Blazor has two different internet hosting fashions — Hybrid, which targets desktops and cell platforms, and Cellular Blazor Bindings, which is experimental and aiming for multi-platform situations akin to iOS and Android, apart from Home windows and macOS.
Irrespective of the runtime model, Blazor apps are created utilizing Razor Parts, typically also referred to as Blazor Parts or simply parts. Every part is a stand-alone piece of a UI-element, usually fashioned by a mixture of HTML code for the web page structure, and a snippet of C# code for the logic and dynamic content material.
The code beneath exhibits a pattern Blazor part, with a counter discipline and a button within the @web page(HTML)
part, the place the logic is within the @code
part:
@web page "/counter"
<h1>Counter</h1>
<p>Present rely: @currentCount</p>
<button class="btn btn-primary" @onclick="IncrementCount">Click on me</button>
@code {
non-public int currentCount = 0;
non-public void IncrementCount()
{
currentCount++;
}
}
The way forward for Blazor with .NET 8
In November 2023, Microsoft will launch the .NET 8 framework, which is at present in preview v7 (see the .Internet workforce’s bulletins right here).
Particularly for Blazor, the next adjustments are on the present roadmap.
Server-side Rendering
Server-side rendering follows the present logic of Razor pages or MVC Purposes, just like ASP.NET Net Varieties beforehand. Razor Pages is a page-based mannequin. UI and enterprise logic considerations are saved separate, however inside the web page.
Razor Pages is the really helpful solution to create new page-based or form-based apps for builders new to ASP.NET Core. It supplies a neater start line than ASP.NET Core MVC. ASP.NET MVC renders UI on the server and makes use of a model-view-controller (MVC) architectural sample.
The MVC sample separates an app into three fundamental teams of parts: fashions, views, and controllers. Consumer requests are routed to a controller. The controller is accountable for working with the mannequin to carry out consumer actions or retrieve outcomes of queries. The controller chooses the view to show to the consumer, and supplies it with any mannequin knowledge it requires. Assist for Razor Pages is constructed on ASP.NET Core MVC.
Due to server-side rendering (SSR), the server generates the HTML code in response to a request from the browser/shopper. The massive profit with SSR is that efficiency will dramatically improve, as there’s no WebAssembly object to be downloaded when loading the app. In contrast with utilizing Razor pages or MVC — which technically does the identical as what we defined within the earlier sentence — builders can profit from Blazor’s component-based structure, which doesn’t actually exist with Razor or MVC. Whereas the component-based method would possibly really feel completely different at first, as soon as we get the cling of it, we see that numerous code duplication from the previous can now be moved right into a reusable Blazor part. Consider buttons, banners, types, tables,and so forth — the place the article stays however the knowledge content material adjustments.
4 fashions in a single
4 fashions in a single seems like the final word improvement resolution. The present fashions (server-side, Wasm, hybrid and cell bindings) are mixed with .NET 8 right into a single method, irrespective of the situation. Due to Blazor, it’s doable to develop wealthy server-based purposes, and client-only apps with Wasm, in addition to cross-platform iOS, Android and macOS apps — all primarily based on the identical Blazor framework.
Streaming rendering
Streaming rendering is one other promising functionality in .NET 8 Blazor, which is the center floor between server-side and client-side rendering. Keep in mind that, with server-side, the complete HTML web page is rendered by the server. Streaming rendering permits the rendering of the static HTML, in addition to placeholders for content material. As soon as the async server-side name is full — which means it could stream the info — the precise HTML web page is up to date by filling within the placeholder objects with the precise knowledge.
Server Facet and WebAssembly
Server Facet and WebAssembly will nonetheless be out there in the identical means they work with the present model of Blazor, however they’ll be extra optimized.
Auto mode
Auto mode is the one I’m personally having most expectations of, and representing the “final” Blazor situation, permitting a mixture of each server-side and WebAssembly in a single.
This situation gives the preliminary web page from the server, which suggests it can load quick. Subsequently, the mandatory objects are downloaded to the shopper, so the subsequent time the web page masses, it’s provided from Wasm. (This model isn’t current within the .NET 8 preview 7 but, so there aren’t extra particulars to share on the time of scripting this.) When you assume that is fairly just like the “4 fashions in a single” method described earlier, know that auto mode is focusing on browser apps, not full desktop or cell platform situations.
Constructing Our First Blazor .NET 8 Net App
Now we’ve got a significantly better understanding of what Blazor is all about, and what’s getting ready for the subsequent launch of .NET 8, let’s stroll by a hands-on situation, the place we’ll create our first .NET 8 Blazor app.
Stipulations
To make this as platform unbiased as doable, the idea is you might have a machine with the next parts:
- Obtain .NET 8 Preview 7 from right here and set up it in your improvement workstation.
- Obtain Visible Studio Code from right here and set up it in your improvement workstation.
- Create a subfolder named
Blazor8sample
. This folder will host the .NET utility.
Constructing the app
With the conditions out of the best way, let’s stroll by the subsequent steps: creating an app utilizing the Blazor template, and working it on our improvement workstation.
-
Open a terminal window, and browse to the
Blazor8sample
folder created earlier. -
Run the next command to validate the .NET model:
dotnet --version
-
Run the next command to get the main points for the brand new Blazor template:
dotnet new Blazor /?
-
Run the next command to create the brand new Blazor App:
dotnet new Blazor -o Blazor8SampleApp
-
Navigate to the subfolder
Blazor8SampleApp
, and runcode .
to open the folder in Visible Studio Code. -
From inside Visible Studio Code, navigate to Terminal within the prime menu, and choose New Terminal (or use Ctrl + Shift + ` shortcut keys on Home windows). This opens a brand new Terminal window inside VS Code.
-
Within the Terminal window, run the next command to launch the Blazor App:
dotnet run .Blazor8SampleApp
-
Navigate to the localhost listening URL (akin to
http://localhost:5211
in my instance). Observe: the port is perhaps completely different in your machine, so examine the URL for the precise port quantity.This opens the working Blazor Net App in your default browser. Open the Climate web page.
-
From inside VS Code, navigate to the
Program.cs
file. The Blazor Net App template is ready up for Server-side rendering of Razor parts by default. InProgram.cs
the decision toAddRazorComponents()
provides the associated providers, after whichMapRazorComponents<App>()
discovers the out there parts and specifies the basis part for the app. -
Whenever you chosen the Climate menu possibility, the web page can have briefly confirmed Loading, after which it rendered the climate knowledge in a desk. That is an instance of the Stream Rendering characteristic as mentioned earlier.
-
From inside VS Code, navigate to the
/Pages/Climate.razor
web page. Open the code view.Discover line 2:
@attribute [StreamRendering(true)]
This enables for the brand new Blazor Stream Rendering characteristic to work.
-
Cease the working app by closing the browser, or press Ctrl + C from the terminal window. Replace the earlier code part to this:
@attribute [StreamRendering(false)]
-
Save the adjustments, and run the app once more by initiating
dotnet run . Blazor8SampleApp.csproj
from the terminal window. -
Browse to the working utility once more by clicking on the
http://localhost:5211
URL, and click on the Climate app. Discover how, this time, there’s no Loading… message proven, but it surely takes just a few seconds for the web page to render and present the precise climate desk.
Abstract
The journey of net utility improvement has developed from static HTML to the dynamic and interactive experiences we take pleasure in (and anticipate!) as we speak. With .NET Blazor, Microsoft has taken a major step in providing builders a strong framework that unifies client-side and server-side improvement paradigms.
As we eagerly anticipate the discharge of .NET Blazor 8, we are able to look ahead to enhanced efficiency, improved tooling, and options akin to server-side in addition to stream rendering, which can proceed to raise the online improvement panorama. Whether or not you’re a seasoned .NET developer or a newcomer to the ecosystem, .NET Blazor opens doorways to constructing next-generation net purposes with the facility of C# and .NET.
FAQs About .NET Blazor
Blazor is an open-source net framework developed by Microsoft that enables builders to construct interactive net purposes utilizing C# and .NET as an alternative of JavaScript. It permits full-stack improvement by working C# code straight within the browser, making it a strong various to conventional JavaScript frameworks.
Blazor consists of two main internet hosting fashions: Blazor WebAssembly and Blazor Server. Blazor WebAssembly runs C# code within the browser, whereas Blazor Server runs C# code on the server and makes use of SignalR to speak with the shopper.
Blazor WebAssembly is a client-side internet hosting mannequin by which your complete Blazor utility is downloaded and executed within the consumer’s browser. This enables Blazor purposes to work offline, however it could have barely slower preliminary load occasions in comparison with Blazor Server.
Blazor Server is a server-side internet hosting mannequin the place the C# code runs on the server and communicates with the shopper utilizing SignalR. It gives real-time capabilities and is appropriate for purposes that require server-side processing and minimal shopper assets.
Sure, Blazor WebAssembly is well-suited for constructing PWAs. It means that you can create net purposes that may be put in on a consumer’s machine and work offline.
Blazor primarily makes use of C# for writing server-side and client-side code. It additionally helps Razor, a syntax for constructing dynamic net pages utilizing C# or HTML. Razor parts are a key a part of Blazor improvement.
[ad_2]